Saturday, 27 April 2024
New Features
On System Settings’ Region & Language page, you can now choose how you’d like storage sizes to be displayed. This means you can change them to the more common MB and GB style, instead of MiB and GiB, for example. Note that the default value has not changed, but you have the option to change it yourself (Méven Car, Plasma 6.1. Link):
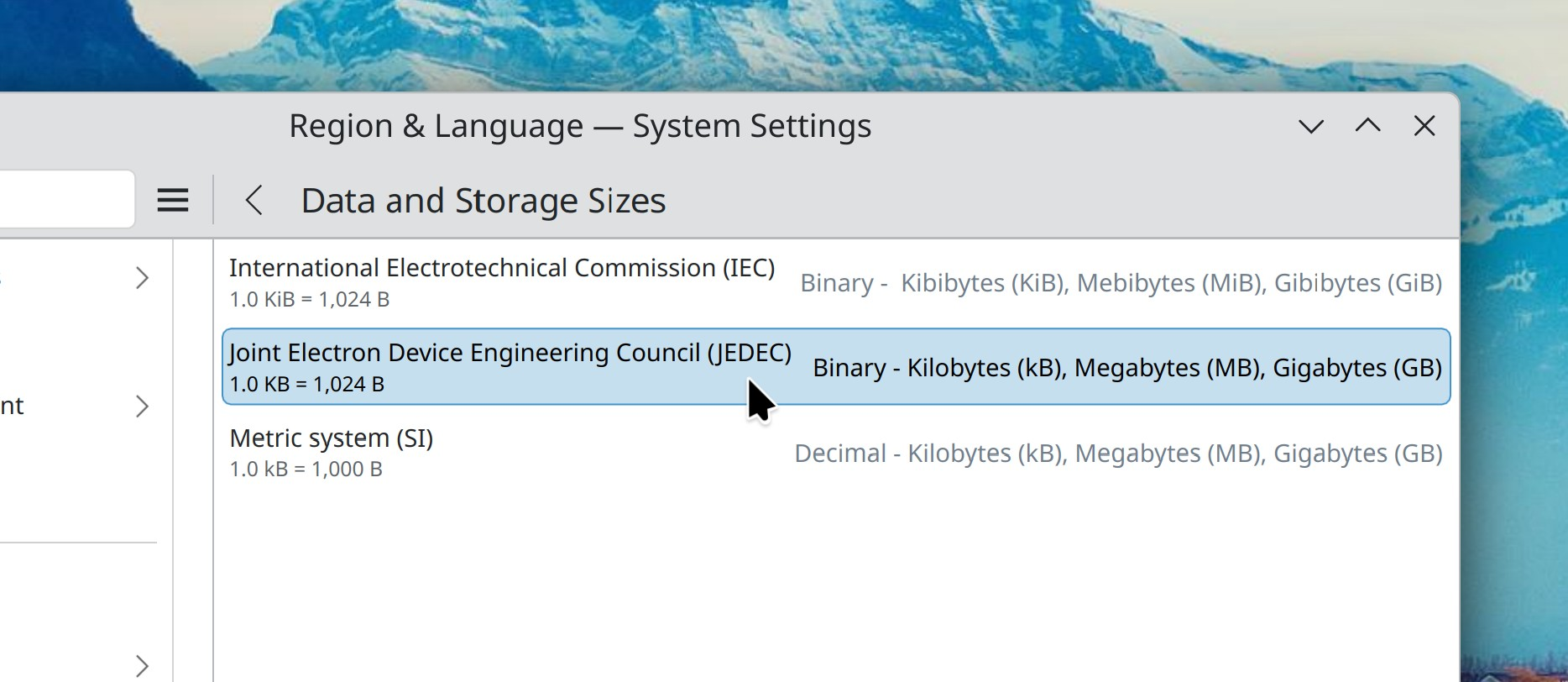
The popular Kirigami.ContextualHelpButton
component now has a QtWidgets counterpart: KContextualHelpButton
! (Felix Ernst, Frameworks 6.2. Link)
UI Improvements
On X11, Spectacle now makes it obvious that screen recording isn’t supported when you try to run it in recording mode using a global shortcut (Noah Davis, Spectacle 24.05. Link)
Plasma’s Vaults widget now appears visible in the active part of the system tray only when a Vault is actually open, bringing the System Tray closer to its platonic ideal of only showing things that are contextually relevant (me: Nate Graham, Plasma 6.1. Link)
Apps inhibiting sleep and screen locking are now shown by their pretty names, not their technical names (Natalie Clarius, Plasma 6.1. Link):
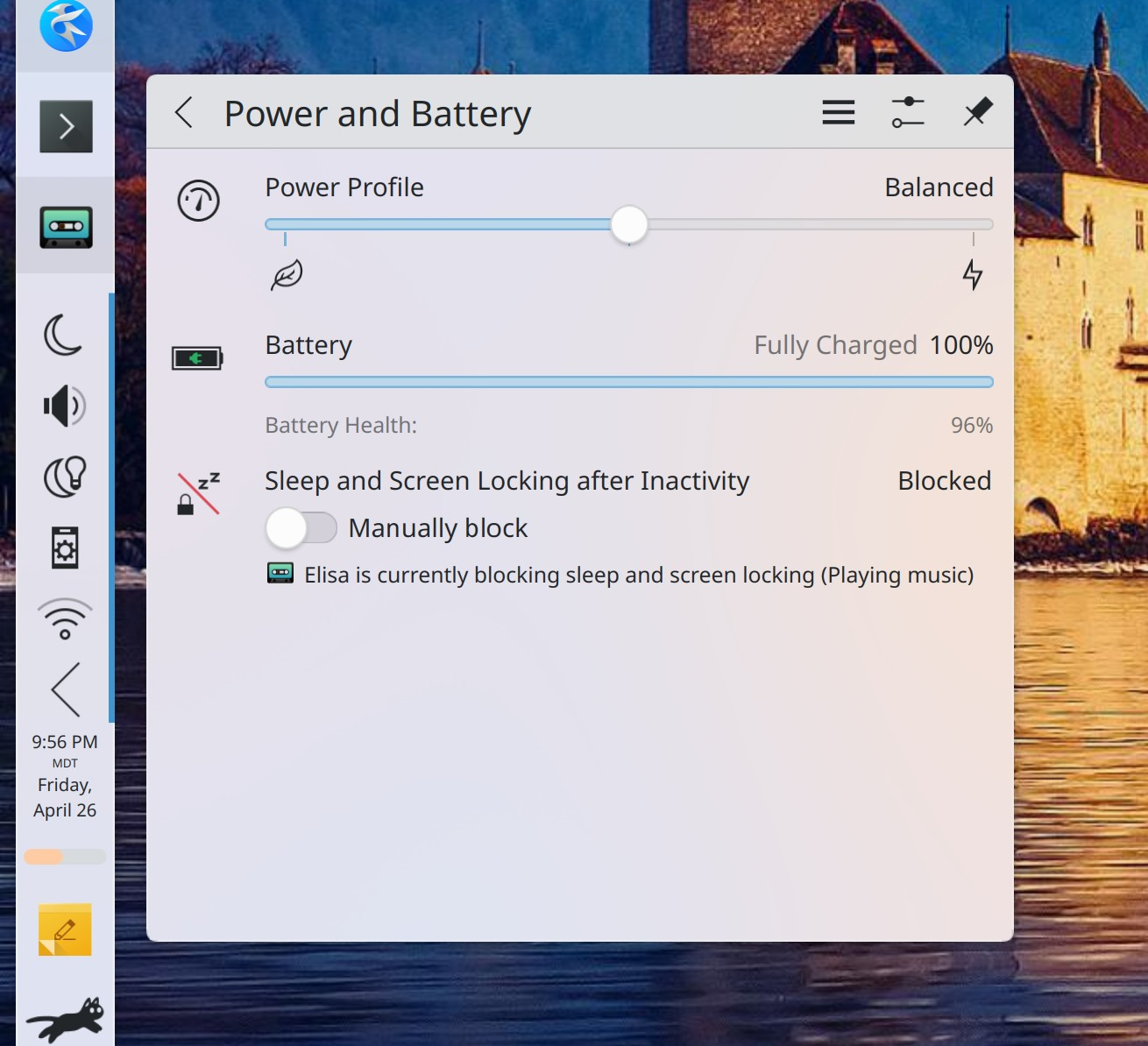
Adopted the new frameless message header style in Discover, as seen when there’s a distro upgrade available (me: Nate Graham, Plasma 6.1. Link):

Bug Fixes
Kate once again works as expected with multiple virtual desktops, opening files in a new window in the current virtual desktop rather than attaching it to an existing instance in a different virtual desktop (Christoph Cullmann, Kate 24.05. Link)
Fixed Spectacle’s multi-monitor screenshots on X11 not working either at all, or well (Vlad Zahorodnii, and Noah Davis, Plasma 6.0.5. and Spectacle 24.05. Link 1 and link 2)
Fixed a semi-common crash in System Monitor when switching to the Applications page (Arjen Hiemstra, Plasma 6.0.5. Link)
When updating the system using Discover, there are no longer gaps in the updates list as items complete and disappear (Ivan Tkachenko, Plasma 6.0.5. Link)
Plasma’s feature to remember whether Bluetooth was on or off last time now works more reliably (Someone going by the pseudonym “Arctic Lampyrid”, Plasma 6.0.5. Link)
Fixed an issue in Plasma that would cause flickering and stuttering with adaptive sync turned on (Xaver Hugl, Plasma 6.0.5. Link)
Floating panels now de-float when a window reaches the correct distance away from them, as opposed to de-floating too early (Yifan Zhu, Plasma 6.0.5. Link)
Fixed multiple issues involved with LPD printer discovery and queue management (Mike Noe, Plasma 6.1. Link)
When you have multiple Brightness and Color widgets (for example, because you have multiple panels each with a System Tray on it), the Night Light portions of the widgets no longer interfere with one another and interact in strange ways (Natalie Clarius, Plasma 6.1. Link)
Other bug information of note:
- 3 Very high priority Plasma bugs (same as last week). Current list of bugs
- 39 15-minute Plasma bugs (up from 38 last week). Current list of bugs
- 116 KDE bugs of all kinds fixed over the last week Full list of bugs
Performance & Technical
Improved Spectacle-s startup speed on Wayland (Noah Davis, Spectacle 24.05. Link)
Improved the scrolling performance of long scrollable views in Discover. This is an area of focus, so expect more to come soon (Aleix Pol Gonzalez, Plasma 6.0.5. Link)
Reduced visual glitchiness when the GPU does a reset, which can happen due to driver bugs, or, with NVIDIA, when the system goes to sleep (Xaver Hugl, Plasma 6.1. Link)
Implemented support for the org.freedesktop.impl.portal.Secret
portal for KWallet, which lets Flatpak apps use it (Nicolas Fella, Frameworks 6.2. Link)
Automation & Systematization
Added some basic GUI tests for Dolphin (Méven Car, link)
Added basic GUI tests for opening Plasma’s Alternate Calendar config page (Fushan Wen, link)
…And Everything Else
This blog only covers the tip of the iceberg! If you’re hungry for more, check out https://planet.kde.org, where you can find more news from other KDE contributors.
How You Can Help
The KDE organization has become important in the world, and your time and labor have helped to bring it there! But as we grow, it’s going to be equally important that this stream of labor be made sustainable, which primarily means paying for it. Right now the vast majority of KDE runs on labor not paid for by KDE e.V. (the nonprofit foundation behind KDE, of which I am a board member), and that’s a problem. We’ve taken steps to change this with paid technical contractors—but those steps are small due to growing but still limited financial resources. If you’d like to help change that, consider donating today!
Otherwise, visit https://community.kde.org/Get_Involved to discover other ways to be part of a project that really matters. Each contributor makes a huge difference in KDE; you are not a number or a cog in a machine! You don’t have to already be a programmer, either. I wasn’t when I got started. Try it, you’ll like it! We don’t bite!