Model/View Drag and Drop in Qt - Part 1
Model/View Drag and Drop in Qt - Part 1
This blog series is all about implementing drag-and-drop in the Qt model/view framework. In addition to complete code examples, you'll find checklists that you can go through to make sure that you did not forget anything in your own implementation, when something isn't working as expected.
At first, we are going to look at Drag and Drop within a single view, to change the order of the items. The view can be a list, a table or a tree, there are very little differences in what you have to do.
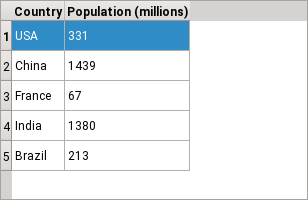
Moving a row in a tableview, step 1
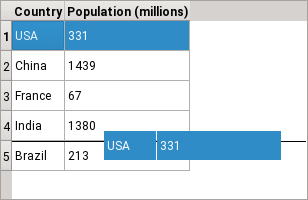
Moving a row in a tableview, step 2
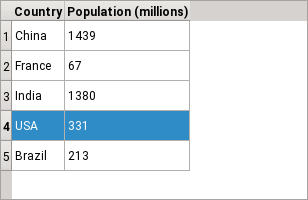
Moving a row in a tableview, step 3
The main question, however, is whether you are using QListView/QTableView/QTreeView on top of a custom item model, or QListWidget/QTableWidget/QTreeWidget with items in them. Let's explore each one in turn.
With Model/View separation
The code being discussed here is extracted from the example. That example features a flat model, while this example features a tree model. The checklist is the same for these two cases.
Setting up the view
☑ Call view->setDragDropMode(QAbstractItemView::InternalMove)
to enable the mode where only moving within the same view is allowed
☑ When using QTableView
, call view->setDragDropOverwriteMode(false)
so that it inserts rows instead of replacing cells (the default is false
for the other views anyway)
Adding drag-n-drop support to the model
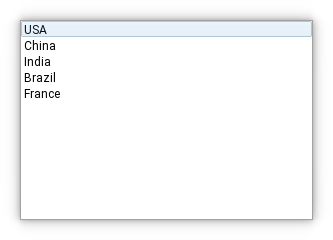
Reorderable ListView
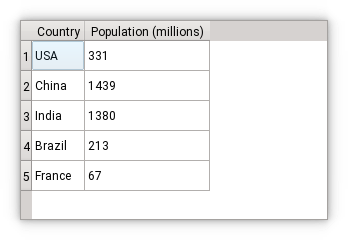
Reorderable TableView
For a model being used in QListView or QTableView, all you need is something like this:
class CountryModel : public QAbstractTableModel
{
~~~
Qt::ItemFlags flags(const QModelIndex &index) const override
{
if (!index.isValid())
return Qt::ItemIsDropEnabled; // allow dropping between items
return Qt::ItemIsEnabled | Qt::ItemIsSelectable | Qt::ItemIsDragEnabled;
}
// the default is "copy only", change it
Qt::DropActions supportedDropActions() const override { return Qt::MoveAction; }
// the default is "return supportedDropActions()", let's be explicit
Qt::DropActions supportedDragActions() const override { return Qt::MoveAction; }
QStringList mimeTypes() const override { return {QString::fromLatin1(s_mimeType)}; }
bool moveRows(const QModelIndex &sourceParent, int sourceRow, int count, const QModelIndex &destinationParent, int destinationChild) override; // see below
};
The checklist for the changes you need to make in your model is therefore the following:
☑ Reimplement flags()
For a valid index, add Qt::ItemIsDragEnabled
and make sure Qt::ItemIsDropEnabled
is NOT set (except for tree models where we need to drop onto items in order to insert a first child). \
☑ Reimplement mimeTypes()
and make up a name for the mimetype (usually starting with application/x-
)
☑ Reimplement supportedDragActions()
to return Qt::MoveAction
☑ Reimplement supportedDropActions()
to return Qt::MoveAction
☑ Reimplement moveRows()
Note that this approach is only valid when using QListView
or, assuming Qt >= 6.8.0, QTableView
- see the following sections for details.
In a model that encapsulates a QVector
called m_data
, the implementation of moveRows
can look like this:
bool CountryModel::moveRows(const QModelIndex &sourceParent, int sourceRow, int count, const QModelIndex &destinationParent, int destinationChild)
{
if (!beginMoveRows(sourceParent, sourceRow, sourceRow + count - 1, destinationParent, destinationChild))
return false; // invalid move, e.g. no-op (move row 2 to row 2 or to row 3)
for (int i = 0; i < count; ++i) {
m_data.move(sourceRow + i, destinationChild + (sourceRow > destinationChild ? 0 : -1));
}
endMoveRows();
return true;
}
QTreeView does not call moveRows
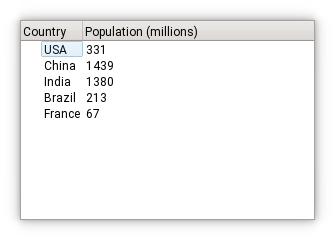
Reorderable treeview
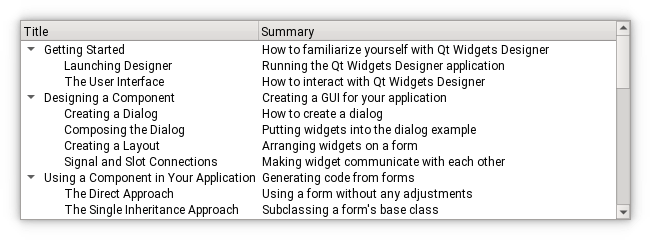
Reorderable treeview with a tree model
QTreeView does not (yet?) call moveRows
in the model, so you need to:
☑ Reimplement mimeData()
to encode row numbers for flat models, and node pointers for tree models
☑ Reimplement dropMimeData()
to implement the move and return false (meaning: all done)
Note that this means a move is in fact an insertion and a deletion, so the selection isn't automatically updated to point to the moved row(s).
QTableView in Qt < 6.8.0
I implemented moving of rows in QTableView
itself for Qt 6.8.0, so that moving rows in a table view is simpler to implement (one method instead of two), more efficient, and so that selection is updated. If you're not yet using Qt >= 6.8.0 then you'll have to reimplement mimeData()
and dropMimeData()
in your model, as per the previous section.
This concludes the section on how to implement a reorderable view using a separate model class.
Using item widgets
The alternative to model/view separation is the use of the item widgets (QListWidget
, QTableWidget
or QTreeWidget
) which you populate directly by creating items.
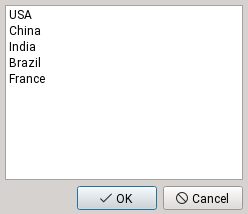
Reorderable QListWidget
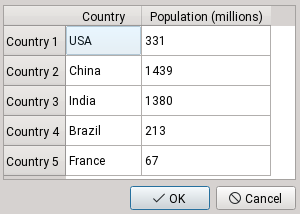
Reorderable QTableWidget
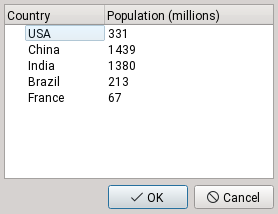
Reorderable QTreeWidget
Here's what you need to do to allow users to reorder those items.
Example code can be found following this link.
Reorderable QListWidget
☑ Call listWidget->setDragDropMode(QAbstractItemView::InternalMove)
to enable the mode where only moving within the same view is allowed
For a QListWidget
, this is all you need. That was easy!
Reorderable QTableWidget
When using QTableWidget
:
☑ Call tableWidget->setDragDropMode(QAbstractItemView::InternalMove)
☑ Call tableWidget->setDragDropOverwriteMode(false)
so that it inserts rows instead of replacing cells
☑ Call item->setFlags(item->flags() & ~Qt::ItemIsDropEnabled);
on each item, to disable dropping onto items
Note: Before Qt 6.8.0, QTableWidget
did not really support moving rows. It would instead move data into cells (like Excel). The example code shows a workaround, but since it calls code that inserts a row and deletes the old one, header data is lost in the process. My changes in Qt 6.8.0 implement support for moving rows in QTableWidget
's internal model, so it's all fixed there. If you really need this feature in older versions of Qt, consider switching to QTableView
.
Reorderable QTreeWidget
When using QTreeWidget
:
☑ Call tableWidget->setDragDropMode(QAbstractItemView::InternalMove)
☑ Call item->setFlags(item->flags() & ~Qt::ItemIsDropEnabled);
on each item, to disable dropping onto items
Conclusion about reorderable item widgets
Of course, you'll also need to iterate over the items at the end to grab the new order, like the example code does. As usual, item widgets lead to less code to write, but the runtime performance is worse than when using model/view separation. So, only use item widgets when the number of items is small (and you don't need proxy models).
Improvements to Qt
While writing and testing these code examples, I improved the following things in Qt 6.8:
- QTBUG-13873 / QTBUG-101475 - QTableView: implement moving rows by drag-n-drop
- QTBUG-69807 - Implement QTableModel::moveRows
- QTBUG-130045 - QTableView: fix dropping between items when precisely on the cell border
- QTBUG-1656 - Implement full-row drop indicator when the selection behavior is SelectRows
Conclusion
I hope this checklist will be useful when you have to implement your own reordering of items in a model or an item-widget. Please post a comment if anything appears to be incorrect or missing.
In the next blog post of this series, you will learn how to move (or even copy) items from one view to another.
The post Model/View Drag and Drop in Qt - Part 1 appeared first on KDAB.